Image coordinates and pixel IDs
Before we consider the task at hand further, let's clarify a few points. First, the raster is a coordinate space where the origin is at the top left corner.
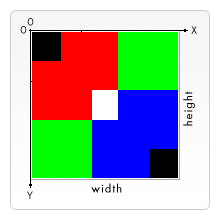
Open the Demo.java
file and update the code as follows:
BufferedImage image = ImageUtility.getBufferedImage("tiny/color.png");
Color[][] pixels = ImageUtility.getPixels(image);
- ImageUtility.printColors(pixels);
+ ImageUtility.printCoordinates(pixels);
I have replaced the printColors
function with the printCoordinates
in the above snippet. Save the changes and run the demo program. You must see the following output.
(0,0) (1,0) (2,0) (3,0) (4,0)
(0,1) (1,1) (2,1) (3,1) (4,1)
(0,2) (1,2) (2,2) (3,2) (4,2)
(0,3) (1,3) (2,3) (3,3) (4,3)
(0,4) (1,4) (2,4) (3,4) (4,4)
This output shows the coordinates ($x$ and $y$ values) of each pixel (cell) in the $5$-by-$5$ color.png
image. Notice the top left corner is the origin. The $x$ increases as you go from left to right on the horizontal axis. The $y$ increases as you go from top to bottom on the vertical axis.
In this mapping, the image width corresponds to the number of columns in the grid and the image height to the number of rows.
According to this coordinate system, we define a unique ID to represent each pixel, as follows: a pixelID
for the pixel at $(x,y)$ is $y \times w + x$ where $w$ is the image width (number of columns).
In the Demo.java
file, replaced the printCoordinates
function with the printPixelIDs
. Save the changes and run the demo program. You must see the following output.
0 1 2 3 4
5 6 7 8 9
10 11 12 13 14
15 16 17 18 19
20 21 22 23 24
This output shows the ID of each pixel (cell) in the $5$-by-$5$ color.png
image. Notice the pixels are labeled with values $0$ to $24$, inclusive, in row-major order.
Resources
These resources are provided for completeness; you do not need to consult any of these for this take-home exam!
- Wikipedia's entry on Coordinate_system.
- Wikipedia's entry on Row- and column-major order.