An Implementation of IndexedList
We are aiming for a very simple implementation of IndexedList
that uses the built-in Java array as internal storage of data.
Exercise Completed the implementation of ArrayIndexedList
/**
* An implementation of IndexedList that takes a default value
* to plaster over the entire data structure.
*/
public class ArrayIndexedList implements IndexedList {
private int[] data;
/**
* Construct an ArrayIndexedList with given size and default value.
*
* @param size the capacity of this list.
* @param defaultValue an integer to plaster over the entire list.
*/
public ArrayIndexedList(int size, int defaultValue) {
// TODO Implement me!
}
@Override
public void put(int index, int value) {
// TODO Implement me!
}
@Override
public int get(int index) {
return 0; // TODO Implement me!
}
@Override
public int length() {
return 0; // TODO Implement me!
}
}
Solution
/**
* An implementation of IndexedList that takes a default value
* to plaster over the entire data structure.
*/
public class ArrayIndexedList implements IndexedList {
private int[] data;
/**
* Construct an ArrayIndexedList with given size and default value.
*
* @param size the capacity of this list.
* @param defaultValue an integer to plaster over the entire list.
*/
public ArrayIndexedList(int size, int defaultValue) {
data = new int[size];
for (int i = 0; i < size; i++) {
data[i] = defaultValue;
}
}
@Override
public void put(int index, int value) {
data[index] = value;
}
@Override
public int get(int index) {
return data[index];
}
@Override
public int length() {
return data.length;
}
}
Notice
-
The
IndexedList
ADT does not specify how it must be implemented. Therefore, the decisions such as using an array internally, having a fixed length, and initializing the array with a default value are made byArrayIndexedList
. -
There is no need to write JavaDoc for methods declared (and documented) in the
IndexedList
interface as one can always see the documentation there.
Here is a class diagram that represents our type hierarchy so far:
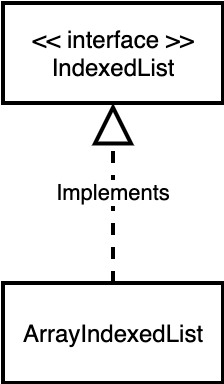
ArrayIndexedList
is our first official data structure in this course! 🎉